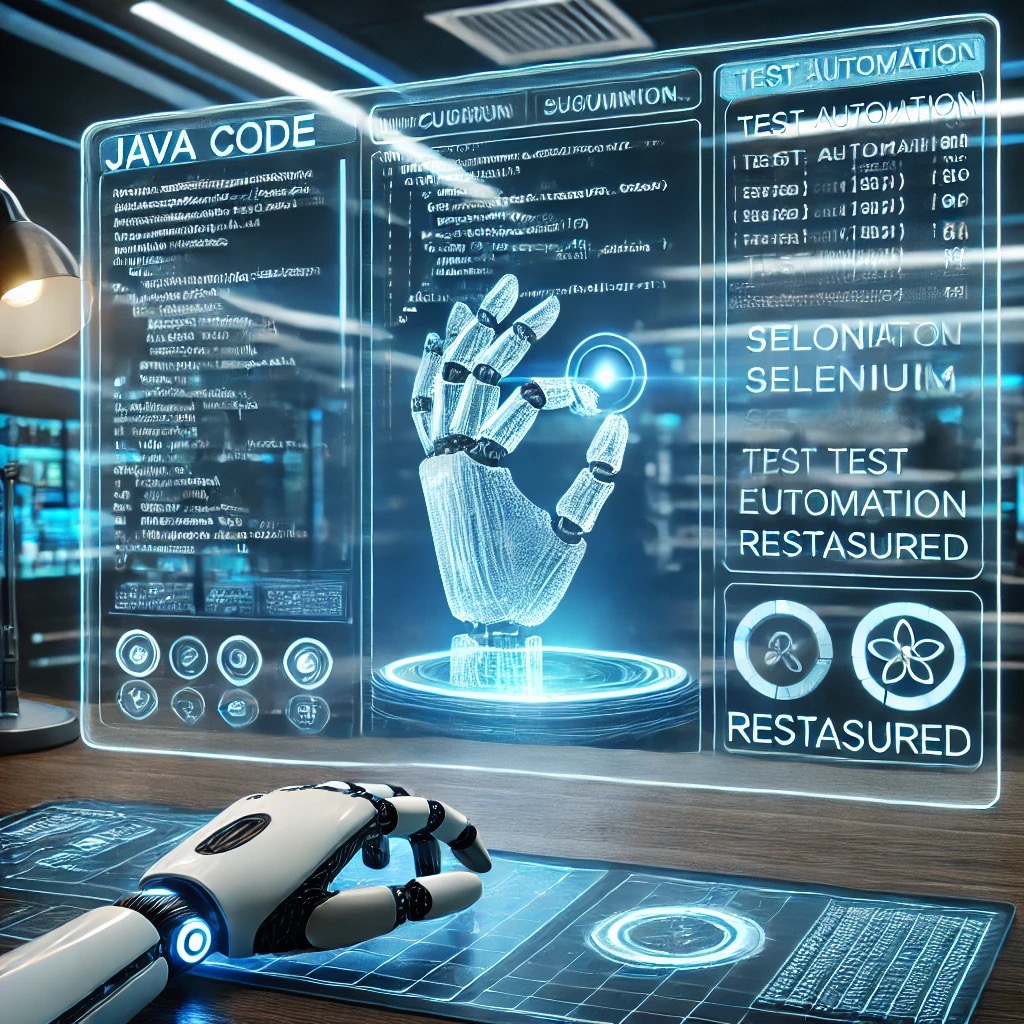
Revisiting My First Test Automation Framework in Java
- Alexander Wahl
- Development , Testing , Automation
- February 28, 2025
Table of Contents
While organizing some of my old GitHub repositories recently, I stumbled upon one of my first serious test automation projects - the Lazarus Test Framework. Looking back at this code brought back memories of my early days in test automation, and I thought it might be interesting to share this journey.
The Birth of Lazarus
Back in 2020, I was working on a project that required extensive web testing across multiple browsers. Manual testing was becoming unsustainable, and I needed a robust, flexible solution that could handle both UI and API testing. That’s when I decided to build Lazarus.
Named after the biblical figure who rose from the dead, the framework was designed to bring tests back to life - making them reliable, maintainable, and easy to write. It was my first comprehensive attempt at creating a test automation framework from scratch.
Technical Foundation
Lazarus was built on a solid stack of Java-based technologies:
- Maven for dependency management and build automation
- Cucumber for behavior-driven development (BDD) and readable test scenarios
- Selenium WebDriver for browser automation
- Rest Assured for API testing
- Log4j for comprehensive logging
- JUnit for test execution and assertions
The framework also supported integration with Appium for mobile testing, though I never fully explored that capability in production.
Architecture Overview
Looking at the codebase now, I can see how I structured it following the Page Object Model pattern, which was (and still is) considered a best practice for UI test automation:
src/
├── test/
├── java/
│ └── lazarus/
│ ├── steps/
│ │ ├── backend/ # API test steps
│ │ ├── pages/ # Page Object Model implementations
│ │ ├── web/ # Web UI test steps
│ │ ├── DriverCreation.java # WebDriver setup
│ │ ├── StepDefinitions.java # Cucumber step definitions
│ │ └── StepHelper.java # Logger and WebDriver initialization
│ └── utilities/ # Helper classes
└── resources/
├── drivers/ # WebDrivers for different OS
├── features/ # Cucumber feature files
├── jenkinsFiles/ # CI/CD integration
└── utilities/
└── TestConstants/ # Test constants
Feature Highlights
BDD with Cucumber
One of the aspects I’m still proud of is how the framework embraced BDD principles. Here’s an example of a feature file I created for testing Wikipedia:
@WikipediaHomePage
Feature: Testing wordings and title on the home page
Scenario Outline: Test web site
Given I am using the browser Chrome
And I go to the main web page "<Web Page>"
Then I start to check that in right top corner I have text "<Text>" and link title "<Title>"
Examples:
| Web Page | Text | Title |
| https://en.wikipedia.org/wiki/Main_Page | Welcome to | Wikipedia |
This approach made tests readable for non-technical stakeholders and served as living documentation.
Page Object Model
The framework implemented a clean separation of concerns through the Page Object Model. For example, here’s how I structured the Wikipedia page object:
// MainPage.java in pages/wiki package
public class MainPage {
private WebDriver driver;
private By welcomeToText = By.xpath("//path/to/element");
private By welcomeTitle = By.xpath("//path/to/title");
public MainPage(WebDriver driver) {
this.driver = driver;
}
public String get_welcome_to() {
return driver.findElement(welcomeToText).getText();
}
public String get_welcome_title() {
return driver.findElement(welcomeTitle).getText();
}
}
And the corresponding step definition:
public class Steps extends StepHelper {
@And("I go to the main web page {string}")
public void i_go_to_the_main_web_page(String url) {
logger.info("The web page, which should be checked, is: " + url);
driver.get(url);
}
@Then("I start to check that in right top corner I have text {string} and link title {string}")
public void iStartToCheckThatInRightTopCornerIHaveTextAndLinkTitle(String text, String title) {
MainPage mainpage = new MainPage(driver);
logger.info("Checking the greeting wording...");
Assert.assertEquals("Welcome to", mainpage.get_welcome_to());
logger.info("Checking the title...");
Assert.assertEquals("Wikipedia", mainpage.get_welcome_title());
}
}
Beautiful HTML Reports
The framework generated comprehensive HTML reports using the cucumber-reporting plugin. These reports included:
- Test execution statistics
- Detailed step-by-step results
- Screenshots for failed tests
- Execution time metrics
Docker Integration
In the testing_ti8m
branch, I added Docker support to make the framework more portable and easier to run in CI/CD environments:
# Run a specific test tag in Docker
docker compose run --rm test mvn test -Dcucumber.options="--tags @CareerTi8mCareerAvailability"
This was a significant improvement that allowed tests to run consistently across different environments.
Common Commands
The framework supported several Maven commands for different testing scenarios:
# Run tests with a specific tag
mvn test -Dcucumber.filter.tags="@Test"
# Run all tests
mvn test
# Clean the project
mvn clean
# Clean, compile, test, and package
mvn clean install
Lessons Learned
Looking back at this project, I can see both the strengths and weaknesses of my approach:
What Worked Well:
- The BDD approach with Cucumber made tests readable and maintainable
- The Page Object Model created a clean separation between test logic and UI interaction
- The reporting system provided clear visibility into test results
- The framework was flexible enough to handle both UI and API testing
What I Would Do Differently:
- Implement better error handling and recovery mechanisms
- Add more robust parallel execution capabilities
- Create a more comprehensive configuration system
- Improve the documentation with more examples
Real-World Example: Handling Captchas on Accenture’s Career Portal
One of the most challenging aspects of web automation is dealing with captchas. While working on Lazarus, I implemented a solution for handling captchas on Accenture’s career portal form.
Video Demonstration
I’ve recorded a video demonstration showing how the framework handles a real-world scenario - filling out Accenture’s careers subscriptions application form and solving the captcha challenge:
The Captcha Challenge
Captchas are deliberately designed to stop automation, making them one of the trickiest obstacles in test automation. For this project, I integrated with an external captcha-solving API service that could process the image and return the text solution.
The video demonstrates how the framework:
- Navigates to Accenture’s career portal
- Fills out the application form with test data
- Captures the captcha image
- Sends it to the external API service
- Receives the solution and inputs it into the form
- Submits the application
This capability made Lazarus particularly valuable for testing protected forms and login pages that would otherwise require manual intervention.
Conclusion
Revisiting Lazarus has been a nostalgic journey. While I’ve moved on to more sophisticated testing approaches since then, this framework served its purpose well and taught me valuable lessons about test automation architecture.
The principles I applied in Lazarus - BDD, Page Object Model, clean separation of concerns - are still relevant today, even as the specific technologies continue to evolve.
For those interested in exploring the code, you can find it on my GitHub repository.
What was your first serious automation framework like? I’d love to hear about your experiences in the comments below!